Event Organiser (& Pro) use a lot of custom hooks. Hooks, if you don’t know what they are, allow a third-party plug-in or a theme to modify the behaviour of WordPress – or, in this case, Event Organiser (& Pro).
As an example, in this tutorial I’ll be demonstrating how you can email the venue when a booking is confirmed for one of their events.
Step 1: Adding an Email for a Venue
There isn’t a native ’email’ field for venues, but you can use the custom fields metabox to add an email custom field for your venue. Below, I’ve given this custom field the name ‘venue_email’, and assigned an email to the venue.
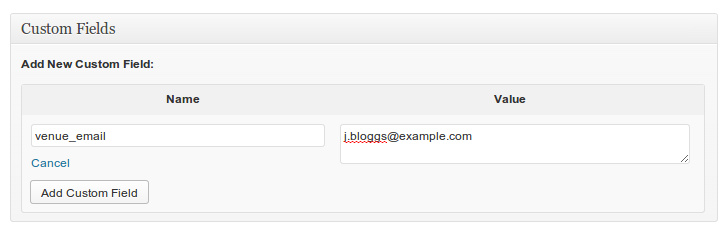
You can assign an email to a venue via the custom fields metabox, and email the venue when a booking is made.
Step 2: Listening for when a Booking is Confirmed
Now on to the coding. Whenever a booking is confirmed the following action is triggered: eventorganiser_booking_confirmed
. This passes the booking ID, so we can look up the booking details – including for which event the booking was made.
add_action( 'eventorganiser_booking_confirmed', 'my_email_venue_on_booking_confirm' );
function my_email_venue_on_booking_confirm( $booking_id ){
//Called when a booking is confirmed
}
Step 3: Email the Venue
From the booking ID we can get the venue ID, and the venue email. That sounds like a lot, but the available API make this a fairly simple procedure:
add_action( 'eventorganiser_booking_confirmed', 'my_email_venue_on_booking_confirm' );
function my_email_venue_on_booking_confirm( $booking_id ){
$event_id = eo_get_booking_meta( $booking_id, 'event_id' );
//If event has avenue
if( $venue_id = eo_get_venue( $event_id ) ){
$venue_email = eo_get_venue_meta( $venue_id, 'venue_email', true );
$venue_name = eo_get_venue_name( $venue_id );
//If the venue has a valid email stored with it:
if( $venue_email = sanitize_email( $venue_email ) ){
//Get email template
$template = eventorganiser_pro_get_option( 'email_template' );
//Set subject
$subject = "A booking was made for your venue";
//Set message
$message = sprintf( "A booking was made for the venue: %s", $venue_name );
//Send the email. This function is a wrapper for wp_mail()
eventorganiser_mail( $venue_email , $subject, $message, null, array(), $template );
}
}
}
Where Should This Code Go?
As always, in a seperate plug-in (ideally). But it will work in your theme’s functions.php
too. See this post for more details.
Resources:
The codex lists all functions available which you can use to retrieve information about the event / booking to include in the mail. Including:
eo_get_booking_meta()
– http://codex.wp-event-organiser.com/function-eo_get_booking_meta.htmleo_get_schedule_start()
– –http://codex.wp-event-organiser.com/function-eo_get_schedule_start.htmleventorganiser_mail()
– http://codex.wp-event-organiser.com/function-eventorganiser_mail.htmleo_get_venue()
– http://codex.wp-event-organiser.com/function-eo_get_venue.htmleo_get_venue_meta()
– http://codex.wp-event-organiser.com/function-eo_get_venue_meta.html
Host Limiting Your Emails?
Most hosts offering shared hosting will limit the number of emails you can sender from your server. The way to get around this is to use SMTP and use an email provider to send the emails. Event Organiser works with WP SMTP a plug-in which allows you to do this. You can find more details here: https://wp-event-organiser.com/pro-features/e-mailing-bookees/#host-limiting-your-emails